@bccampus/react-composite
Base React component to create a composite components, like Listbox,TreeView, with selection and expansion management
Installation
Usage
Kim Tornado
Software Developer
Aria Patel
Data Scientist
Elena Rodriguez
UX/UI Designer
Liam Carter
Marketing Specialist
Sophie Williams
Financial Analyst
Adrian Smith
Project Manager
Mia Johnson
Graphic Designer
Jessica Brown
Customer Support Specialist
Raj Gupta
Network Engineer
Emily Chang
Human Resources Manager
Focus behavior
Composite
component applies different focus movement and selection strategies based on the layout (Keyboard navigation).
Currently, it provides focus behaviors for vertical lists, horizontal lists, and layout grids.
Options
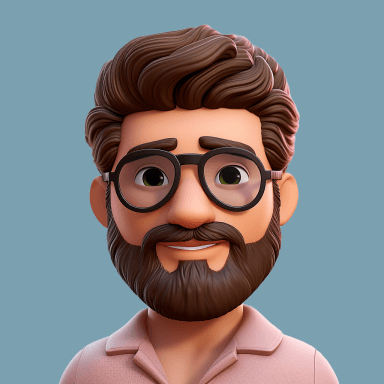
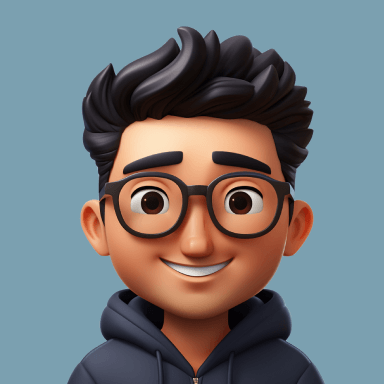
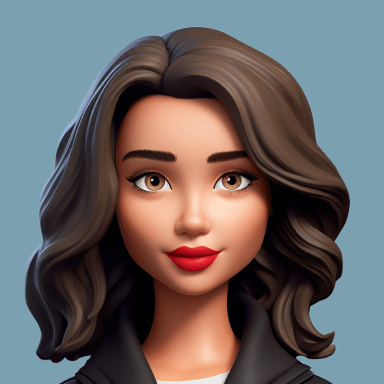
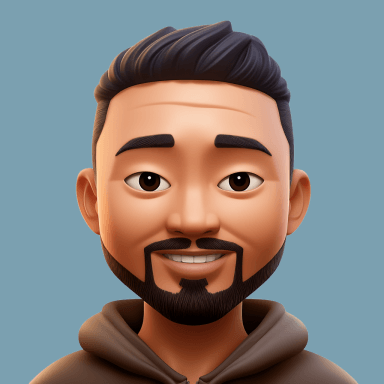
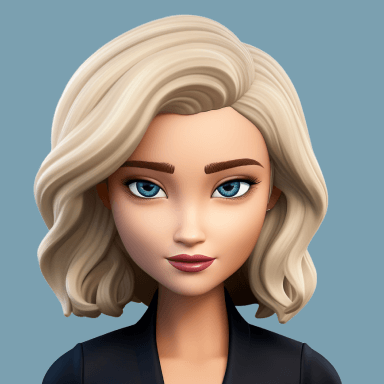
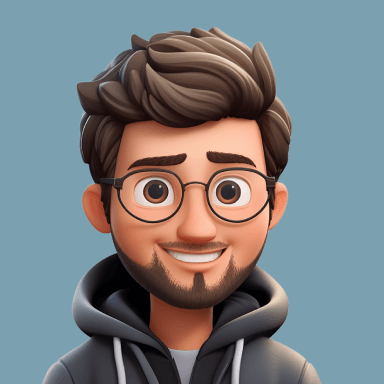
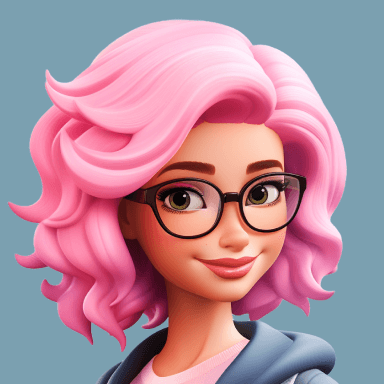
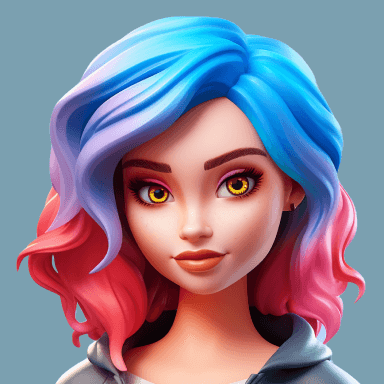
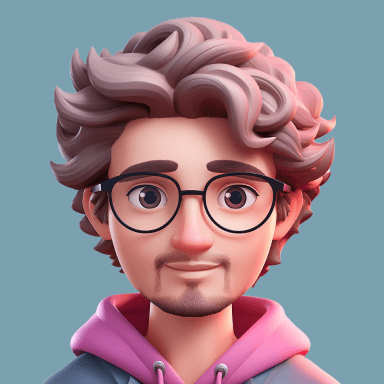
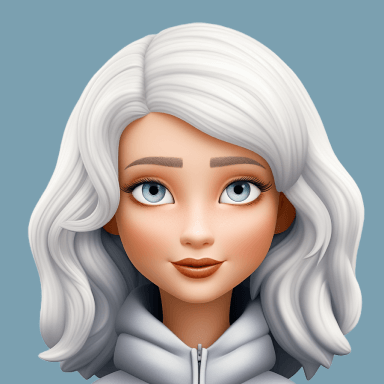
Defining custom keys
By default, Composite
uses id
or key
property of items as their unique identifier.
If your items do not contain any of these properties, you can use getItemKey
property to define a custom key.
Selection state
Expansion state
Introduction to Algorithms, Fourth Edition
Preface
I Foundations
II Sorting and Order Statistics
III Data Structures
IV Advanced Design and Analysis Techniques
Introduction
14 Dynamic Programming
15 Greedy Algorithms
16 Amortized Analysis
V Advanced Data Structures
VI Graph Algorithms
VII Selected Topics
VIII Appendix: Mathematical Background
Bibliography
Index
Disabled items
You can disable items in a Composite
component by setting the disabledKeys
option.
Disabled options can not be selected and the focus movement skips these items.
Additionaly, aria-disabled
HTML attribute is set to true
for these items, which can be used for custom styling.
Composite context
CompositeContext
provides two boolean values multiple
and selectionMode
to identify the selection type and
whether the component in the selection mode. selectionMode
changes only if selectionOptions.trackSelectioMode
is set to true.
The following example shows how items can change based on these values.
If nothing is selected (selectionMode: false
), an overlay and checkbox is shown only if an item is hovered.
After an item is selected (selectionMode: true
), all items render their overlays.
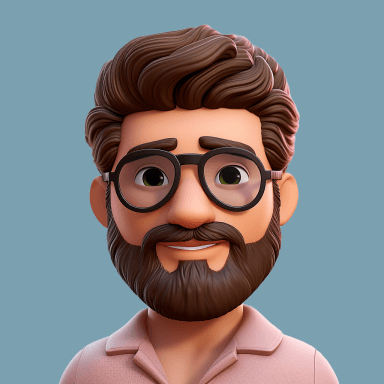
Kim Tornado
Software Developer
Passionate about creating efficient and scalable software solutions. Experienced in full-stack development with a focus on web applications. Enjoys tackling complex coding challenges and learning new technologies.
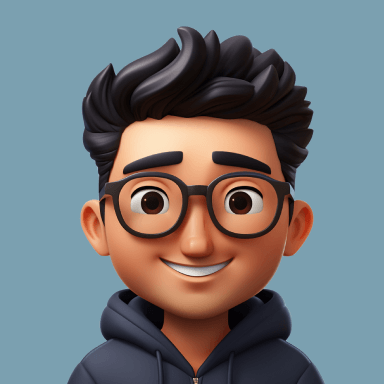
Aria Patel
Data Scientist
Data enthusiast with expertise in statistical analysis and machine learning. Proficient in using Python and R for data manipulation and modeling. Excited about uncovering meaningful insights from complex datasets.
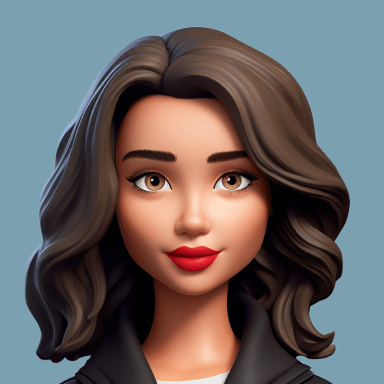
Elena Rodriguez
UX/UI Designer
Creative designer with a passion for crafting engaging user experiences. Skilled in creating intuitive interfaces that blend aesthetics with functionality. Enjoys staying updated on design trends and user-centered design principles.
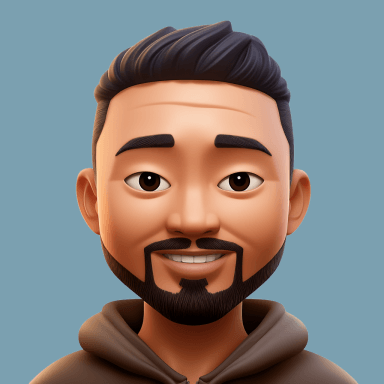
Liam Carter
Marketing Specialist
Results-driven marketer with a focus on digital marketing strategies. Experienced in campaign management, SEO, and social media marketing. Enjoys analyzing market trends and developing creative marketing initiatives.
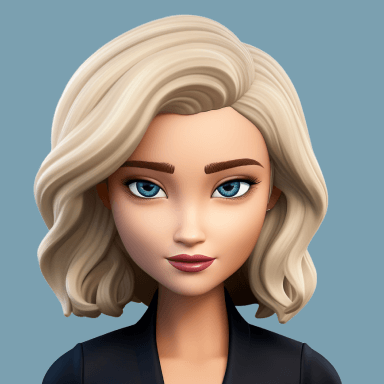
Sophie Williams
Financial Analyst
Detail-oriented financial analyst with expertise in financial modeling and forecasting. Proficient in analyzing financial statements and providing insights for informed decision-making. Enjoys working with numbers and solving financial puzzles.
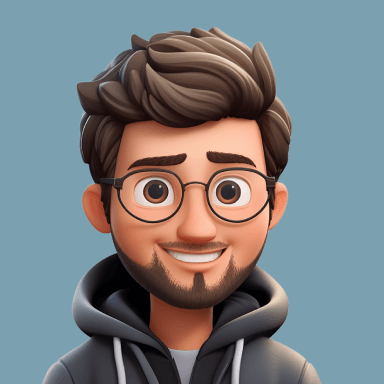
Adrian Smith
Project Manager
Seasoned project manager with a track record of successful project delivery. Skilled in team coordination, resource allocation, and risk management. Enjoys leading cross-functional teams and ensuring projects are completed on time and within budget.
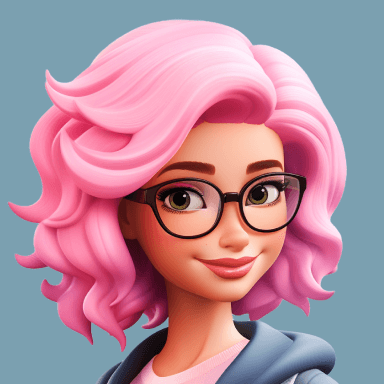
Mia Johnson
Graphic Designer
Creative graphic designer with a passion for visual storytelling. Proficient in Adobe Creative Suite and experienced in creating visually stunning designs for both print and digital media. Enjoys bringing ideas to life through impactful visuals.
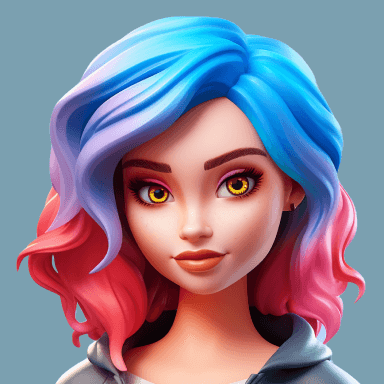
Jessica Brown
Customer Support Specialist
Dedicated customer support specialist with excellent communication and problem-solving skills. Experienced in addressing customer inquiries, resolving issues, and ensuring customer satisfaction. Enjoys providing top-notch support and building positive customer relationships.
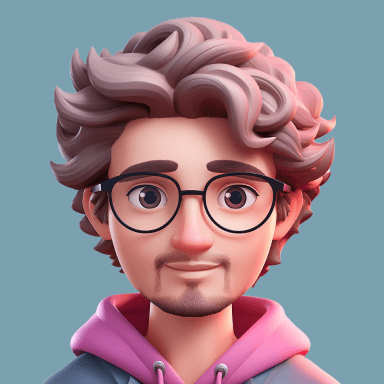
Raj Gupta
Network Engineer
Experienced network engineer with expertise in designing and implementing robust network infrastructure. Skilled in troubleshooting network issues and optimizing performance. Enjoys staying updated on the latest networking technologies and security protocols.
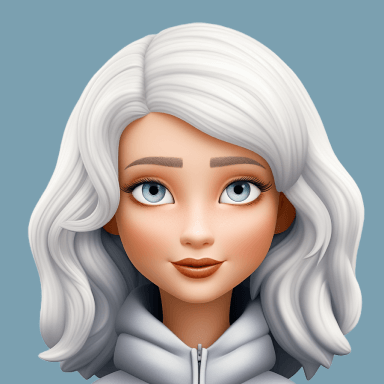
Emily Chang
Human Resources Manager
Strategic human resources manager with a focus on talent acquisition and employee development. Skilled in workforce planning, performance management, and HR policy implementation. Enjoys fostering a positive work environment and supporting organizational growth.
Keyboard navigation
Vertical/Horizontal List
Focus:
Down Arrow
/Right Arrow
: Moves focus to the next option.- If focus is on the last item and
focusOptions.loop: true
, focus moves to the first item.
- If focus is on the last item and
Up Arrow
/Left Arrow
: Moves focus to the previous option.- If focus is on the first item and
focusOptions.loop: true
, focus moves to the last item.
- If focus is on the first item and
Page Down
: Moves focus downfocusOptions.pageSize
number of items. If focus is in the last item of the list, focus does not move.Page Up
: Moves focus upfocusOptions.pageSize
number of items. If focus is in the first item of the list, focus does not move.Home
: Moves focus to first option.End
: Moves focus to last option.
Selection:
-
Space
: changes the selection state of the focused option. -
Control + A
: Selects all options in the list. If all options are selected, it unselect all options.In a single-select list, selection also move with focus when
selectionOptions.followFocus
options is settrue
.In a multi-select list, focus movements with
Shift
modifier, selects contiguous items from the most recently selected item to the focused item. For example,Shift + Home
selects the focused option and all options up to the first option, and moves focus to the first option.
Layout Grid
Focus:
Right Arrow
: Moves focus one cell to the right.- If focus is on the right-most cell in the row and
focusOptions.moveToNextRow: true
, focus moves to the first cell in the following row.focusOptions.moveToNextRow: false
andfocusOptions.loop: true
, focus moves to the first cell in the same row.focusOptions.moveToNextRow: false
andfocusOptions.loop: false
, focus does not move.
- If focus is on the last cell in the grid and
focusOptions.loop: true
, focus moves to the first cell in the grid; otherwise, focus does not move.
- If focus is on the right-most cell in the row and
Left Arrow
: Moves focus one cell to the left.- If focus is on the left-most cell in the row and
focusOptions.moveToNextRow: true
, focus moves to the last cell in the previous row.focusOptions.moveToNextRow: false
andfocusOptions.loop: true
, focus moves to the first cell in the same row.focusOptions.moveToNextRow: false
andfocusOptions.loop: false
, focus does not move.
- If focus is on the first cell in the grid and
focusOptions.loop: true
, focus moves to the last cell in the grid; otherwise, focus does not move.
- If focus is on the left-most cell in the row and
Down Arrow
: Moves focus one cell down.- If focus is on the bottom cell in the column and
focusOptions.moveToNextColumn: true
, focus moves to the top cell in the following column.focusOptions.moveToNextColumn: false
andfocusOptions.loop: true
, focus moves to the top cell in the same column.focusOptions.moveToNextColumn: false
andfocusOptions.loop: false
, focus does not move.
- If focus is on the bottom cell in the last column and
focusOptions.loop: true
, focus moves to the first cell in the grid; otherwise, focus does not move.
- If focus is on the bottom cell in the column and
Up Arrow
: Moves focus one cell up.- If focus is on the top cell in the column and
focusOptions.moveToNextColumn: true
, focus moves to the bottom cell in the previous column.focusOptions.moveToNextColumn: false
andfocusOptions.loop: true
, focus moves to the bottom cell in the same column.focusOptions.moveToNextColumn: false
andfocusOptions.loop: false
, focus does not move.
- If focus is on the first cell in the grid and
focusOptions.loop: true
, focus moves to the bottom cell in the last column; otherwise, focus does not move.
- If focus is on the top cell in the column and
Page Down
: Moves focus downfocusOptions.pageSize
number of rows. If focus is in the last row of the grid, focus does not move.Page Up
: Moves focus upfocusOptions.pageSize
number of rows. If focus is in the first row of the grid, focus does not move.Home
: moves focus to the first cell in the row that contains focus.End
: moves focus to the last cell in the row that contains focus.Control + Home
: moves focus to the first cell in the first row.Control + End
: moves focus to the last cell in the last row.
Selection:
-
Space
: Changes the selection state of the focused option. -
Control + A
: Selects all options in the list. If all options are selected, it unselect all options.In a single-select grid, selection also move with focus when
selectionOptions.followFocus
options is settrue
.In a multi-select grid, focus movements with
Shift
modifier, selects contiguous items from the most recently selected item to the focused item. For example,Control + Shift + Home
selects the focused option and all options up to the first option in the grid, and moves focus to the first option.